Friday, June 19, 2009
C# How to convert byte arry to string
How to a byte array to a string? The solution is using the 'GetString()' method from the ASCIIEncoding.ASCII object. Be carrefull with this method, it may cause problems if you try to convert something other than English, such as Chinese and other asian languages. In this case, you have to use specific ASCII encoding for that language.
For example, you probably want to use System.Text.Encoding.GetEncoding(1251).GetString(b);
Here, '1251' stands for English. You can choose your own codepage for Encoding or Decoding.
Method
byte[] data = ... ; //whatever it is here
string text1 = System.Text.ASCIIEncoding.ASCII.GetString(data);//Let the ASCIIEncoding handle the convertion
string text2 = System.Text.Encoding.GetEncoding(1251).GetString(data);// 1251 stands for English
string text3 = System.Text.Encoding.GetEncoding(28591).GetString(data);// 28591 stands for Latin
string text4 = System.Text.Encoding.GetEncoding("GB18030").GetString(data);// "GB18030" stands for Simplified Chinese
Thursday, June 11, 2009
C# How to beep from your computer
How to make a beep sound from your computer?
Although you can use any sound library to work for you, there is a simple way to make just a beep sound. This becomes useful especially when you are running a program for a long time and you want to be notified when any certain condition reaches.
Thanks to Microsoft, we can achieve this in one line of code with the build-in methods.
Method
Console.Beep();//No parameter
Console.Beep(250, 250);//Parameter: int frequency, int duration
Wednesday, May 13, 2009
C# Convert string to an array of bytes
How to convert a string or a string array into a double array?
The .NET does not provide any method for us to call directly(if you found it, please let me know :D). However this is easy to implement within just a couple lines of code.
Method
//Convert 1 string to an array of bytes
public static byte[] ConvertToBytes(string str)
{
byte[] ans = new byte[str.Length];
for (int i = 0; i < ans.Length; i++)
{
ans[i] = Convert.ToByte(str[i]);
}
return ans;
}
Tuesday, May 5, 2009
How to disable a TabPage in the TabControl
Scenario
The component TabControl in the Visual Studio IDE does not allow people to
disable a certain TabPage within the TabControl. I have Googled for a long time
and there is not any real solution regarding this issue. However, some people put a
customized TabControl which allows you to disable the TabPage. I believe not
everyone wants to download and try the third party software or plug-in.
Method
I'm going to talk about a 'solution' which is more like a trick to solve this problem.
For example, let's create a TabControl and it has 2 TabPages. Now before
dragging any other objects from the tool list, drag a Panel object and drop into
each one of those TabPages. Set those three Panels' Dock property to Fill so
that the panel will be maximized within its TabPage. Now you will probably guess
what I'm trying to tell you. Yes, you put all other objects on top of the panel.
Then you just disable the panel if you want to disable a TabPage. Because the
Panel is the Parent of the other components, the panel and its children will be
disabled if we try to disable the panel itself.
Details
Let's have a look at how this works.
First, create a TabControl and drop a Panel onto one of the tab pages.
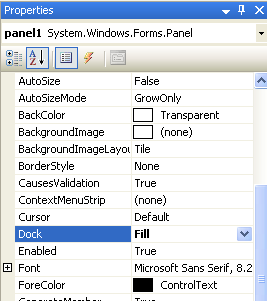
Now, set the Dock to Fill for the panel
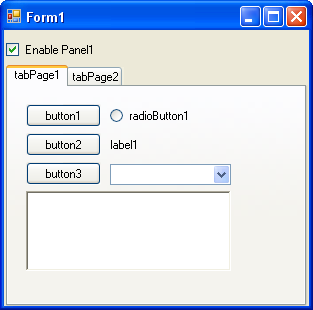
Add some components to the tabPage1. Actually they are on top of the
panel1. Run the sample application without checking the 'Enable Panel1'
checkbox.

The code implementation for the checkbox. Just for testing.
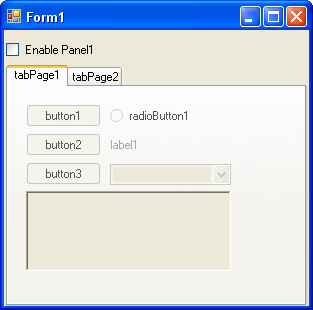
The effect shows the tabPage1 is 'disabled' once the checkbox is unchecked.
Summary
This solution might not be perfect to everyone, but I believe it will help some
people like me to solve a problem by using a simple solution. Remember the KISS
principle? This is all about it!
Sunday, May 3, 2009
Windows 7's Hardware Requirement
The Windows 7 RC version has been released on Apr 30, 2009 for Technet subscribers only. On May 5 everyone should be able to get it for free. Here is the hardware requirement for installing Windows 7 RC on your computer. We can see that it is about the same as Windows Vista, but Windows 7 is 3 years later than Windows Vista.
Windows 7 Hardware Requirements:
·CPU 1GHz(32 bits OR 64 bits)。
·1GB Memory(32 Bits);2GB(64 Bits)。
·16GB Hard Drive(32 Bits);20GB Hard Drive(64 Bits)。
·Support WDDM 1.0 or higher DirectX 9Friday, May 1, 2009
Convert File Size To Readable Format
When we use "Windows + E" to browse files, we will see the file size of each file under the "Details" mode. Every file has a file size, but by default all file sizes are displayed in one unit 'KB'. This makes people difficult to make sense if the file size is greater than 10MB, because you will see a lot of numeric digits before the dot symbol.
Method
The following table is not magic and the issue mentioned above is not hard to solve. Here is a way to convert the file size into human readable format under normal circumstance.
1 KB = 1024 Byte = 1024 Byte
1 MB = 1024 KB = 1024 * 1024 Byte = 1048576 Byte
1 GB = 1024 MB = 1024 * 1024 KB = 1024 * 1024 * 1024 Byte = 1073741824 Byte
To convert the file size you retrieve from the original "File.Length" method, we can use the above table to check the byte count.
Code

Conclusion
As you see, after calling the "GetFileSize" method, it will return a converted format of file size string. The implementation is only a few if statements.
You can copy & modify the above code for your convenience.
Tuesday, April 28, 2009
C# Convert string to double array
How to convert a string or a string array into a double array?
The .NET does not provide any method for us to call directly. However this is easy to implement within just a couple lines of code.
Method
//str is the input string that contains a sequence of numbers
string[] arrString = str.Split(new char[] { ' ' });//you can define your own way to split the string
double[] arrDouble = new double[arrString.Length];
for (int i = 0; i < arrString.Length; i++)
{
arrDouble[i] = double.Parse(arrString[i]);
}
C# Cross-Thread Operations
Cross-Thread Operations
Many of you may have seen the cross-thread exception when dealing with Cross-Thread operations. For example, if you try to call a Form function from a separate thread, you will get an error message similar to:
Cross-thread operation not valid: Control 'Form1' accessed from a thread other than the thread it was created on.
That is, you cannot manA Cross-Thread operation in C# is a call that accesses components from a different thread. Handling corss-thread is a requirement starting with .NET Framework 2.0. We are going to discuss how to make cross-thread calls between controls.
Delegates
The answer is simply to use delegates to invoke methods that use cross-thread operations. Using delegates is an elegant way to call methods that are from other threads.
Methods
The example is quite simple. Let's create a blank winForm application. Then create a second thread called secondThread. Within the secondThread, we try to change the text of 'Form1'. The above exception message will pop up if the following stuff is not implemented.
InvokeRequired
The key for cross-thread calls is in the InvokeRequired property. When cross-threading will be required, the property will be true. This is a way to let you know wether you are in a cross-thread issue or not. You can then use delegates to invoke the method properly:
if (this.InvokeRequired)
{
DoSthDelegate aDelegate = new DoSthDelegate(DoSth); //delegate
this.Invoke(aDelegate); //call the method
}
else
{
//Your code here
}
Others
For more about delegates, refer to http://msdn.microsoft.com/en-us/library/ms173171(VS.80).aspx
For more about Invoke method, refer to http://msdn.microsoft.com/en-us/library/zyzhdc6b.aspx
Tuesday, April 21, 2009
MCTS for C# Developers

The Microsoft Certified Technology Specialist (MCTS) certifications enable professionals to target specific technologies and to distinguish themselves by demonstrating in-depth knowledge and expertise in their specialized technologies. An MCTS is consistently capable of implementing, building, troubleshooting, and debugging a particular Microsoft technology.

_512.png)
You can go to the MCP website and order your own MCTS certification. It should be mailed to you within 1 ~ 2 months. Here is a sample certification you will get. Obviously, "YOUR NAME" means your real name will be placed there.

Exam 70-536
Microsoft .NET Framework - Application Development Foundation more
Training Kit download link
Exam 70-526
Microsoft .NET Framework 2.0 – Windows-Based Client Development more
Training Kit download link
Exam 70-528
Microsoft .NET Framework 2.0 - Web-Based Client Development more
Training Kit download link
Thursday, April 16, 2009
Simple Messenger - A C# MSN Messenger-like Chat Application
Introduction
This is a simple MSN Messenger like chat application using socket programming. It allows the users to send and receive messages by running two Simple Messengers.
You can read this article from my blog: http://hantou.blogspot.com/.
There are two specific features other than the regular MSN Messenger:
- '
Hex
' - TRUE: The data will be displayed in Hex format.
- FALSE: The data will be displayed in regular text.
- '
No print on receiving
' - TRUE: The received data will not be printed on the textbox.
- FALSE: The received data will be printed on the textbox.
Background
One day when I was working on socket programming, I needed to monitor data transmission. Although Visual Studio already has a debugger for the user to monitor the data, I still needed a third application to cache the data and take a look at it. This comes with the idea for the Simple Messenger. You can use this program as a chat application when you run two simple Messengers. If you connect your computer to a device, another software, a web application, etc., you will be able to send/receive data via one Simple Messenger application. In my case, I just connect my computer to a device which has Ethernet connection and I need to monitor the data sent by the device during the run time. The main purpose of this article here is to share this program with you regarding socket programming and multiple threads.
KeyValuePair
A helper class wraps a Socket
and a byte
array.

public class KeyValuePair
{
public Socket socket;
public byte[] dataBuffer = new byte[1];
}
Overview
There are two main classes named Server.cs and Client.cs standing for Server mode and Client mode respectively. Note, the scenario is that only one server and one client are connected. This means that if you try to open one server and multiple clients then I cannot promise anything. The one-to-many case is not implemented and this is beyond the main issue that is being discussed here.
Connection
The connection is slightly different between the Server Mode and the Client Mode.
First look at the Server Mode:

public void Connect(string ipAddr, string port)
{
server = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPEndPoint ipLocal = new IPEndPoint(IPAddress.Any, Convert.ToInt32(port));
server.Bind(ipLocal);//bind to the local IP Address...
server.Listen(5);//start listening...
// create the call back for any client connections...
server.BeginAccept(new AsyncCallback(OnClientConnect), null);
}
The server needs to watch for the connection to see if there is any client trying to connect to it.

public void OnClientConnect(IAsyncResult asyn)
{
try
{
if (server != null)
{
tempSocket = server.EndAccept(asyn);
WaitForData(tempSocket);
server.BeginAccept(new AsyncCallback(OnClientConnect), null);
}
}
/* ... */
}
Before the server socket receives any data, we must prepare for it ahead. Here the KeyValuePair
object is imported as a parameter.

public void WaitForData(Socket soc)
{
try
{
if (asyncCallBack == null)
asyncCallBack = new AsyncCallback(OnDataReceived);
KeyValuePair aKeyValuePair = new KeyValuePair();
aKeyValuePair.socket = soc;
// now start to listen for incoming data...
aKeyValuePair.dataBuffer = new byte[soc.ReceiveBufferSize];
soc.BeginReceive(aKeyValuePair.dataBuffer, 0, aKeyValuePair.dataBuffer.Length,
SocketFlags.None, asyncCallBack, aKeyValuePair);
}
/* ... */
}
Now look at the Client Mode:

public void Connect(string ipAddr, string port)
{
client = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPEndPoint ipe = new IPEndPoint(IPAddress.Parse(ipAddr), Convert.ToInt32(port));
client.Connect(ipe);
clientListener = new Thread(OnDataReceived);
isEndClientListener = false;
clientListener.Start();
}
Data Receive
Since the AsyncCallback
is used for the server, receiving the data will be different.
For the Server Mode

KeyValuePair aKeyValuePair = (KeyValuePair)asyn.AsyncState;
//end receive...
int iRx = 0;
iRx = aKeyValuePair.socket.EndReceive(asyn);
if (iRx != 0)
{
byte[] recv = aKeyValuePair.dataBuffer;
}
For the Client Mode

byte[] recv = new byte[client.ReceiveBufferSize]; //you can define your own size
int iRx = client.Receive(recv );
if (iRx != 0)
{
//recv should contain the received data.
}
Data Send
Sending the data is as easy as calling the Send()
method that comes with the Socket
. E.g.

soc.Send(dataBytes);//'soc' could either be the server or the client
Error Handling
In Server's receive block, you might want to handle the SocketException
with ErrorCode == 10054
. This error code indicates the connection reset for peers. Refer to MSDN.

if (e.ErrorCode == 10054)//Connection reset,
// http://msdn.microsoft.com/en-us/library/ms740668(VS.85).aspx
{
/* ... */
}
Points of Interest
Every time I used the MSN Messenger to chat with my friends, I was worried if my conversation would be recorded by 'MSN'. Now I'm happy and 'safe' to use my own chat application to chat with my friends, er... within a local network. However, the main benefit of this program is that I learned how to play with the Ethernet and sockets. I hope this will benefit you as well.
For more information about me, please visit my blog: hantou.blogspot.com.
History
- 2nd July, 2008 - First version
- 16th April, 2009 - Updated article downloads and images
- 20th April, 2009 - Updated article
Wednesday, April 15, 2009
Requirement Analysis

I found this interesting picture picture yesterday and strongly feel that it exactly describe what I'm working on everyday! We all have stress from work, family, friends, etc. This funny cartoon is a way to relax myself just because someone has point out what I'm thinking daily.
I actually found this cartoon from an IT forum. I search the picture on the Google and find that it has a full version of the 'Requirements Analysis' story. Here is the link for you interest: The Project Cartoon. There are up to 18 cell-pictures you can choose and make your own cartoon.
Requirements analysis in systems engineering and software engineering, encompasses those tasks that go into determining the needs or conditions to meet for a new or altered product, taking account of the possibly conflicting requirements of the various stakeholders, such as beneficiaries or users. -- Wiki
Sunday, April 12, 2009
8 Ways to Optimize Your C# Application 2/2
Ways to optimize C# code in .NET development
5. "try catch" vs "if else"
The "try catch" block is used to catch exceptions that are beyond our controls, such as connection problem. The "if else" is one of the basic logic which is being used in both programming and reality. The "try catch" block helps to avoid error-prone calls or any errors that might slow down the application. According to the 'KISS' principle, using "try catch" to keep code "simple", "clean" better than using "if else" statements. However, we should refactor our source code to require less "try catch" or "if else" statements. That should be the main concentrate of code optimization.
6. Replace divisions
Although this issue is not as common as above, you could benifit from it if you are playing with numeric operations such as multiplication, division. The C# language is relatively slow when it comes to division operations. There is an article written by "rob tillaart" from CodeProject: http://www.codeproject.com/KB/cs/FindMulShift.aspx. He introduces an alternative way called "Multiply Shift" in C# to optimize the performance.
7. Using sealed classes when there isn't any derived class
The sealed modifier is primarily used to prevent unintended derivation, but it also enables certain run-time optimizations. In particular, because a sealed class is known to never have any derived classes, it is possible to transform virtual function member invocations on sealed class instances into non-virtual invocations. -- From "gicio" in 'Performance Optimization in C#'
8. 'for' Loop vs. 'foreach' Loop
Again, this is from "gicio" in 'Performance Optimization in C#'.
This code costs tooooo much:
foreach(DataRow currentDataRow in currentDataTable.Rows)
{
newDataTable.ImportRow(currentDataRow);
}
this code runs over 300 % faster (depends on rows count):
DataRow[] allRows = currentDataTable.Select();
foreach (DataRow currentDataRow in allRows)
{
newDataTable.ImportRow(currentDataRow);
}
Conclusion
As you can see these are very simple C# code optimizations and yet they can have a powerful impact on the performance of your application. If you know any other useful C# code optimization ideas, please let me know and I will add them to the list here.
8 Ways to Optimize Your C# Application 1/2
Thursday, April 9, 2009
8 Ways to Optimize Your C# Application 1/2
1. StringBuilder: knowing when to use
The main difference between string and StringBuilder is that using the StringBuilder to modify a string without creating a new object can boost performance when concatenating many strings in a loop. If you have a loop that will make modification to a single string for many iterations, then a StringBuilder class is much faster than a string type. However, if you just want to append/insert something to a string, then a StringBuilder will be over power. In this case, a simple string type will work well.
2. Strings Compare: Non-Case Sensitive
When we compare two strings, usually we use the built-in equals() method for the comparison. In case of ignoring the cases, people use ToLower() or ToUpper() methods. However, ToLower() or ToUpper() are bottlenecks in performance. Here is a way to increase your applications: use string.Compare(str1, str2, true) == 0; for comparing two strings ignoring cases. If str1 and str2 are equal ignoring cases then result will return 0.
3. Strings Compare: "string.Empty "
It is normal to see people use .equals("") for comparing an empty string. A popular practice is that checking a string's length to be 0 is faster than comparing it to an empty string. Using string.Empty will not make anysignificant performance improvement, but bringing you the readable optimization.
4. Using "List<>" instead of using "ArrayList"
Both List<> and ArrayList can store objects within the same list. The difference is that ArrayList can store multiple types of objects while List<> has to specify one type of object. Also, when you extract the object from the list, the List<> does not need to cast the variable to a proper type while ArrayList has to. Therefore, if
you are keeping the same type of variables in one ArrayList, you can gain a performance boost by using List<> instead.
(to be continue...)
8 Ways to Optimize Your C# Application 2/2
Tuesday, April 7, 2009
Add a property to user control
Known issues:
Assume that we create a winform project and a user control which contains a button only. This user control is called "MyUserButton.cs". We want to add a custom property called "TreeView" that should appear on the "Properties" panel.
Detail:
1. In the Solution Explorer panel, right click on your user control "MyUserButton.cs" file and select "View Code".
2. In the class "MyUserButton.cs", create a private variable "treeView" with type "TreeView".
private TreeView treeView;
3. Then type the following code beneath the variable declarations from last step:
public TreeView MyTreeView
{
get { return treeView; }
set { treeView = value; }
}
4. Save the file and add the "MyUserButton" control to the main window. On the Properties grid panel we can find a property named "TreeView" that is what we just created.
Summary
There are some more advance features about adding a property to the user control. Here is just a simple example that giving you a brief idea on it. For more information, the article "How To Add a Custom Font Property to a User Control" is also useful.